如何使用Python OpenCV混合图像?
在这篇文章中,我们将提供一些使用OpenCV的示例。
在OpenCV中混合图像我们将提供一个逐步的示例,说明如何使用Python OpenCV混合图像。下面我们展示了目标图像和滤镜图像。
目标图像
滤镜图像
import cv2
# Two images
img1 = cv2.imread('target.jpg')
img2 = cv2.imread('filter.png')
# OpenCV expects to get BGR images, so we will convert from BGR to RGB
img1 = cv2.cvtColor(img1, cv2.COLOR_BGR2RGB)
img2 = cv2.cvtColor(img2, cv2.COLOR_BGR2RGB)
# Resize the Images. In order to blend them, the two images
# must be of the same shape
img1 =cv2.resize(img1,(620,350))
img2 =cv2.resize(img2,(620,350))
# Now, we can blend them, we need to define the weight (alpha) of the target image
# as well as the weight of the filter image
# in our case we choose 80% target and 20% filter
blended = cv2.addWeighted(src1=img1,alpha=0.8,src2=img2,beta=0.2,gamma=0)
# finally we can save the image. Now we need to convert it from RGB to BGR
cv2.imwrite('Blending.png',cv2.cvtColor(blended, cv2.COLOR_RGB2BGR))
在OpenCV中处理图像我们将展示如何使用Python中的OpenCV应用图像处理。
如何模糊影像import cv2
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
img = cv2.imread('panda.jpeg')
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
blurred_img = cv2.blur(img,ksize=(20,20))
cv2.imwrite("blurredpanda.jpg", blurred_img)
如何申请Sobel Operator你可以在Wikipedia上查看Sobel Operator:https://en.wikipedia.org/wiki/Sobel_operator也可以开始尝试一些过滤器。让我们应用水平和垂直Sobel。img = cv2.imread('panda.jpeg',0)
sobelx = cv2.Sobel(img,cv2.CV_64F,1,0,ksize=5)
sobely = cv2.Sobel(img,cv2.CV_64F,0,1,ksize=5)
cv2.imwrite("sobelx_panda.jpg", sobelx)
cv2.imwrite("sobely_panda.jpg", sobely)
如何对图像应用阈值我们还可以对图像进行二值化。img = cv2.imread('panda.jpeg',0)
ret,th1 = cv2.threshold(img,100,255,cv2.THRESH_BINARY)
fig = plt.figure(figsize=(12,10))
plt.imshow(th1,cmap='gray')
OpenCV中的人脸检测我们将讨论如何使用OpenCV应用人脸检测。我们通过一个实际的可重现的示例来直截了当。逻辑如下:我们从URL(或从硬盘)获取图像。我们将其转换为numpy array,然后转换为灰度。然后通过应用适当的**CascadeClassifier,**我们获得了人脸的边界框。最后,使用PIllow(甚至是OpenCV),我们可以在初始图像上绘制框。import cv2 as cv
import numpy as np
import PIL
from PIL import Image
import requests
from io import BytesIO
from PIL import ImageDraw
# I have commented out the cat and eye cascade. Notice that the xml files are in the opencv folder that you have downloaded and installed
# so it is good a idea to write the whole path
face_cascade = cv.CascadeClassifier('C:\opencv\build\etc\haarcascades\haarcascade_frontalface_default.xml')
#cat_cascade = cv.CascadeClassifier('C:\opencv\build\etc\haarcascades\haarcascade_frontalcatface.xml')
#eye_cascade = cv.CascadeClassifier('C:\opencv\build\etc\haarcascades\haarcascade_eye.xml')
URL = "https://images.unsplash.com/photo-1525267219888-bb077b8792cc?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=1050&q=80"
response = requests.get(URL)
img = Image.open(BytesIO(response.content))
img_initial = img.copy()
# convert it to np array
img = np.asarray(img)
gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray)
# And lets just print those faces out to the screen
#print(faces)
drawing=ImageDraw.Draw(img_initial)
# For each item in faces, lets surround it with a red box
for x,y,w,h in faces:
# That might be new syntax for you! Recall that faces is a list of rectangles in (x,y,w,h)
# format, that is, a list of lists. Instead of having to do an iteration and then manually
# pull out each item, we can use tuple unpacking to pull out individual items in the sublist
# directly to variables. A really nice python feature
#
# Now we just need to draw our box
drawing.rectangle((x,y,x+w,y+h), outline="red")
display(img_initial)
最初的图像是这样的:
然后在绘制边界框后,我们得到:
如我们所见,我们设法正确地获得了四个面,但是我们还发现了窗后的“鬼”……裁剪脸部以分离图像我们还可以裁剪脸部以分离图像for x,y,w,h in faces:
img_initial.crop((x,y,x+w,y+h))
display(img_initial.crop((x,y,x+w,y+h)))
例如,我们得到的第一张脸是:
注意:如果你想从硬盘读取图像,则只需键入以下三行:# read image from the PC
initial_img=Image.open('my_image.jpg')
img = cv.imread('my_image.jpg')
gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
OpenCV中的人脸检测视频这篇文章是一个实际示例,说明了我们如何将OpenCV]与Python结合使用来检测视频中的人脸。在上一篇文章中,我们解释了如何在Tensorflow中应用对象检测和OpenCV中的人脸检测。通常,计算机视觉和对象检测是人工智能中的热门话题。例如考虑自动驾驶汽车,该自动驾驶汽车必须连续检测周围的许多不同物体(行人,其他车辆,标志等)。如何录制人脸检测视频在以下示例中,我们将USB摄像头应用到人脸检测,然后将视频写入.mp4文件。如你所见,OpenCV能够检测到面部,并且当它隐藏在手后时,OpenCV会丢失它。import cv2
# change your path to the one where the haarcascades/haarcascade_frontalface_default.xml is
face_cascade = cv2.CascadeClassifier('../DATA/haarcascades/haarcascade_frontalface_default.xml')
cap = cv2.VideoCapture(0)
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# MACOS AND LINUX: *'XVID' (MacOS users may want to try VIDX as well just in case)
# WINDOWS *'VIDX'
writer = cv2.VideoWriter('myface.mp4', cv2.VideoWriter_fourcc(*'XVID'),25, (width, height))
while True:
ret, frame = cap.read(0)
frame = detect_face(frame)
writer.write(frame)
cv2.imshow('Video Face Detection', frame)
# escape button to close it
c = cv2.waitKey(1)
if c == 27:
break
cap.release()
writer.release()
cv2.destroyAllWindows()
计算机视觉代码的输出只需几行代码即可通过动态人脸检测录制该视频。如果你运行上面的代码块,你将获得类似的视频。
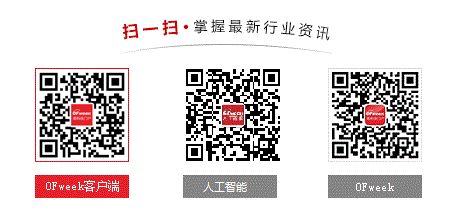
最新活动更多
-
3月27日立即报名>> 【工程师系列】汽车电子技术在线大会
-
4月30日立即下载>> 【村田汽车】汽车E/E架构革新中,新智能座舱挑战的解决方案
-
5月15-17日立即预约>> 【线下巡回】2025年STM32峰会
-
即日-5.15立即报名>>> 【在线会议】安森美Hyperlux™ ID系列引领iToF技术革新
-
5月15日立即下载>> 【白皮书】精确和高效地表征3000V/20A功率器件应用指南
-
5月16日立即参评 >> 【评选启动】维科杯·OFweek 2025(第十届)人工智能行业年度评选
发表评论
请输入评论内容...
请输入评论/评论长度6~500个字
暂无评论
暂无评论